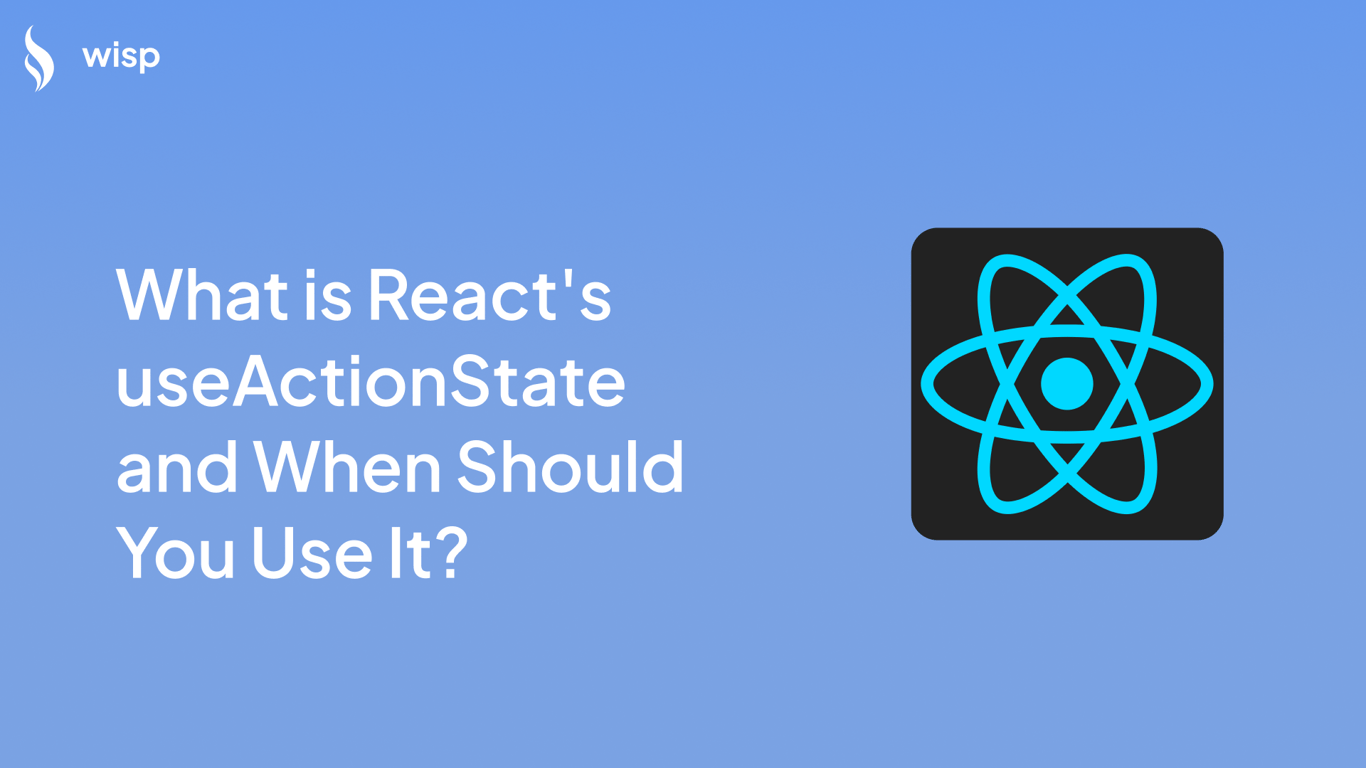
You've been working with React forms and state management, and suddenly you notice there's this new hook called useActionState
. But wait - wasn't there something called useFormState
before? And why are there different import sources? If you're feeling confused about these React hooks, you're not alone.
Many developers have expressed frustration about the transition from useFormState
to useActionState
, especially when it comes to understanding their differences and knowing when to use each one. Today, we'll clear up this confusion and show you exactly how to leverage useActionState
effectively in your React applications.
Understanding useActionState
useActionState
is a React hook available in the Canary releases that helps you manage state updates based on action results - particularly useful in form submissions and interactive components. It was previously known as useFormState
, but was renamed to better reflect its true purpose: managing action states rather than just form states.
Here's what makes it special:
const [state, formAction, isPending] = useActionState(fn, initialState, permalink?);
The hook provides three essential pieces:
A current state value
A form action function
A boolean indicating if the action is pending
One crucial detail that often trips developers up is the import source. As one developer noted:
"I see only that the first one is imported from react: import { useActionState } from "react"; And the other is imported from react-dom: import { useFormState } from "react-dom";"
This distinction is important because useActionState
is the newer, recommended approach, while useFormState
is now deprecated. The move to include it in the core React package (rather than react-dom) signals its broader applicability beyond just form handling.
Key Benefits of useActionState
Broader Applicability: Unlike its predecessor,
useActionState
isn't limited to form contexts. It can track the state of any action, making it more versatile.Immediate Feedback: It provides real-time state updates based on action results, enhancing user experience.
Server Component Integration: It works seamlessly with frameworks supporting React Server Components, enabling faster state updates without waiting for JavaScript execution.
Clearer Intent: The rename from
useFormState
touseActionState
better communicates its purpose - tracking action states rather than just form states.
Practical Examples
Let's look at some real-world examples of how to implement useActionState
effectively.
Basic Counter Example
Here's a simple counter implementation that demonstrates the core functionality:
import { useActionState } from 'react';
async function increment(previousState, formData) {
return previousState + 1;
}
function StatefulCounter() {
const [count, formAction, isPending] = useActionState(increment, 0);
return (
<form>
<p>Current count: {count}</p>
<button formAction={formAction} disabled={isPending}>
{isPending ? 'Incrementing...' : 'Increment'}
</button>
</form>
);
}
Shopping Cart Implementation
Here's a more practical example showing how to handle shopping cart interactions:
import { useActionState } from 'react';
function AddToCartForm({ productId, productName }) {
const [status, formAction, isPending] = useActionState(
async (prevState, formData) => {
try {
await addToCart(productId);
return { success: true, message: 'Added to cart!' };
} catch (error) {
return {
success: false,
message: 'Failed to add item. Please try again.'
};
}
},
null
);
return (
<form>
<h3>{productName}</h3>
<button
formAction={formAction}
disabled={isPending}
>
{isPending ? 'Adding...' : 'Add to Cart'}
</button>
{status && (
<p className={status.success ? 'success' : 'error'}>
{status.message}
</p>
)}
</form>
);
}
Common Pitfalls and Solutions
1. Import Confusion
❌ Wrong way:
import { useFormState } from 'react-dom'; // Deprecated
✅ Correct way:
import { useActionState } from 'react'; // Current recommended approach
2. State Management Outside Forms
A common misconception is thinking these hooks are only for forms. As one developer pointed out:
"useFormState tracks the state of an action, not a form, which can be used outside of
elements."
Best Practices for Using useActionState
1. State Initialization
Always provide a meaningful initial state that matches your expected data structure:
// ❌ Poor initialization
const [state, action] = useActionState(handleSubmit);
// ✅ Good initialization
const [state, action] = useActionState(handleSubmit, {
status: 'idle',
data: null,
error: null
});
2. Error Handling
Implement robust error handling to provide clear feedback:
function SubmitForm() {
const [state, formAction] = useActionState(
async (prevState, formData) => {
try {
const result = await submitData(formData);
return { status: 'success', data: result };
} catch (error) {
return {
status: 'error',
error: error.message || 'An unexpected error occurred'
};
}
},
{ status: 'idle' }
);
return (
<form>
{/* Form fields */}
<button formAction={formAction}>Submit</button>
{state.status === 'error' && (
<div className="error-message">{state.error}</div>
)}
</form>
);
}
3. Loading States
Take advantage of the isPending parameter to show loading states:
function SubmitButton({ formAction, isPending }) {
return (
<button
formAction={formAction}
disabled={isPending}
className={isPending ? 'loading' : ''}
>
{isPending ? 'Processing...' : 'Submit'}
</button>
);
}
When to Use useActionState
Use useActionState
when you need to:
Handle Form Submissions: Perfect for managing form submission states and providing feedback.
Track Async Operations: Ideal for monitoring the status of asynchronous actions.
Provide Immediate Feedback: When you need to update UI based on action results without page refreshes.
Integrate with Server Components: Especially useful in frameworks that support React Server Components.
Advanced Usage and Considerations
Working with Multiple Actions
When dealing with multiple actions in a single component, keep them organized and separate:
function ProductManager() {
const [deleteState, deleteAction] = useActionState(handleDelete, null);
const [updateState, updateAction] = useActionState(handleUpdate, null);
return (
<div>
<form>
<button formAction={deleteAction}>Delete</button>
{deleteState?.message && (
<p className="delete-message">{deleteState.message}</p>
)}
</form>
<form>
<button formAction={updateAction}>Update</button>
{updateState?.message && (
<p className="update-message">{updateState.message}</p>
)}
</form>
</div>
);
}
Integration with Other Hooks
useActionState
works well with other React hooks:
function DataForm() {
const [formState, formAction] = useActionState(handleSubmit, null);
const [localState, setLocalState] = useState('');
useEffect(() => {
if (formState?.success) {
setLocalState(''); // Reset local state after successful submission
}
}, [formState]);
return (
<form>
<input
value={localState}
onChange={(e) => setLocalState(e.target.value)}
/>
<button formAction={formAction}>Submit</button>
</form>
);
}
Conclusion
useActionState
represents a significant improvement in React's state management capabilities, especially for handling action-based state updates. Its transition from useFormState
might have caused some initial confusion, but the rename better reflects its true purpose and broader applicability.
Remember these key points:
Import from 'react', not 'react-dom'
Use it for any action state management, not just forms
Take advantage of the isPending state for better UX
Implement proper error handling
Consider using it with Server Components for optimal performance
By following these guidelines and understanding the proper use cases, you can leverage useActionState
to create more responsive and user-friendly React applications.
Additional Resources
Remember, as React continues to evolve, staying updated with the latest best practices and understanding the reasoning behind changes like the transition from useFormState
to useActionState
will help you build better applications.