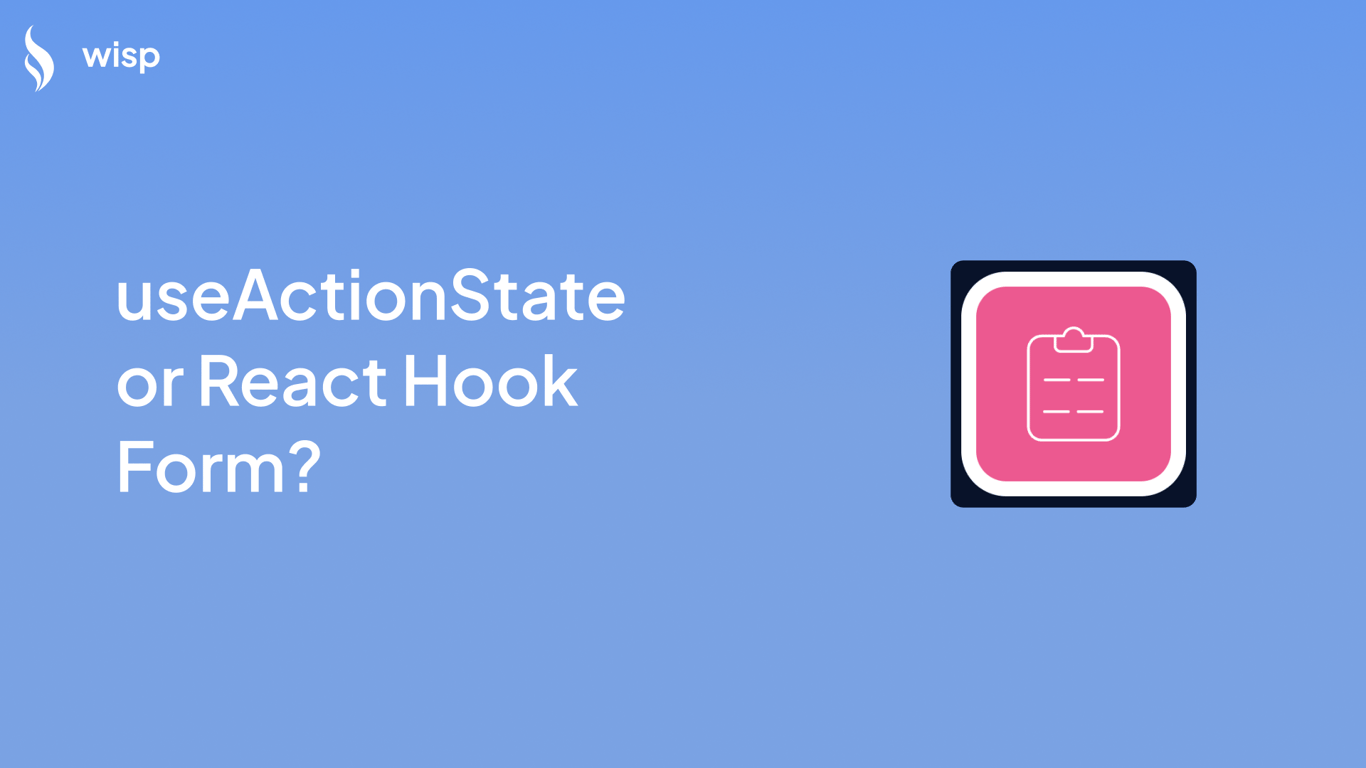
You've just started building a form in your React application, and you're faced with a crucial decision: should you use React's new useActionState
hook or stick with the battle-tested React Hook Form library? As you browse through developer forums and GitHub discussions, you see passionate debates about server-side validation latency, client-side validation needs, and performance implications.
The confusion is real - especially when you're trying to balance modern React features with practical development needs. You might be wondering if adding another dependency like React Hook Form is worth it, or if React's native solution could handle your form requirements effectively.
Let's dive deep into both approaches to help you make an informed decision that won't come back to haunt your project later.
Understanding useActionState
React's useActionState
is a new addition in version 19, designed specifically for managing component state based on form action results. It's particularly powerful when working with React Server Components, offering a native solution for handling form submissions and state updates.
Here's a simple example of useActionState
in action:
import { useActionState } from "react";
async function handleSubmit(previousState, formData) {
// Process form submission
return { success: true, message: "Form submitted!" };
}
function LoginForm() {
const [state, formAction, isPending] = useActionState(handleSubmit, {});
return (
<form>
<input name="username" type="text" />
<input name="password" type="password" />
<button formAction={formAction}>
{isPending ? "Submitting..." : "Submit"}
</button>
{state.message && <p>{state.message}</p>}
</form>
);
}
The hook provides three key elements:
A state value that updates based on form submissions
A formAction function to handle the submission
An isPending boolean to track loading states
While this approach seems straightforward, developers on Reddit have reported challenges with its reliance on server-side validation, which can lead to noticeable latency in user feedback. As one developer noted, "The lack of client-side validation in useActionState can result in a sluggish user experience, especially when dealing with form validations."
Enter React Hook Form
React Hook Form has established itself as a robust solution for form management in React applications. It's designed with performance in mind, focusing on minimizing re-renders and working with uncontrolled components.
Here's how you might implement the same login form using React Hook Form:
import { useForm } from "react-hook-form";
function LoginForm() {
const {
register,
handleSubmit,
formState: { errors, isSubmitting }
} = useForm();
const onSubmit = async (data) => {
// Handle form submission
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input
{...register("username", {
required: "Username is required"
})}
/>
{errors.username && <p>{errors.username.message}</p>}
<input
type="password"
{...register("password", {
required: "Password is required",
minLength: {
value: 8,
message: "Password must be at least 8 characters"
}
})}
/>
{errors.password && <p>{errors.password.message}</p>}
<button disabled={isSubmitting}>
{isSubmitting ? "Submitting..." : "Submit"}
</button>
</form>
);
}
The immediate benefits become apparent:
Instant client-side validation
Built-in error handling
Form state management without additional boilerplate
Type-safe validation when combined with Zod
Many developers prefer this approach because it provides immediate feedback to users without waiting for server responses. As one developer mentioned, "React Hook Form with Zod gives you the best of both worlds - client-side validation for quick feedback and type safety for robust data handling."
Making the Right Choice
The decision between useActionState
and React Hook Form isn't just about personal preference - it's about choosing the right tool for your specific needs. Let's break down when each option shines:
When to Choose useActionState
Simple Forms with Server-Side Logic
When your forms primarily interact with server components
For basic data submission without complex validation requirements
When you want to minimize external dependencies
Server-First Architecture
If your application heavily relies on server-side processing
When you're building with frameworks that emphasize server components
For scenarios where client-side JavaScript should be minimal
Framework Integration
When working with newer React features and patterns
If you're using Next.js or similar frameworks that leverage server actions
When you need tight integration with React's latest capabilities
When to Choose React Hook Form
Complex Form Requirements
Forms with multiple fields and interdependent validations
When immediate user feedback is crucial
For applications with sophisticated form logic
Performance-Critical Applications
When minimizing re-renders is a priority
For forms that require real-time validation
In cases where network latency could impact user experience
Type Safety and Validation
When using TypeScript and requiring type-safe form handling
If you need to integrate with validation libraries like Zod
For projects requiring strict data validation rules
Developer experiences show that React Hook Form often emerges as the preferred choice for complex applications. As one developer noted, "The combination of React Hook Form and Zod has become our go-to solution for handling complex forms with validation requirements."
Best Practices and Recommendations
Based on extensive developer feedback and real-world implementations, here are some best practices to consider:
1. Evaluate Your Form Complexity
Before choosing either solution, assess your form requirements:
How many fields do you need to manage?
What type of validation is required?
Do you need real-time feedback?
2. Consider Your Architecture
Your application's architecture should influence your choice:
Are you building a server-first application?
How important is client-side performance?
What's your approach to handling form submissions?
3. Implementation Tips
For useActionState:
// Combine with server-side validation
async function handleSubmit(previousState, formData) {
const data = Object.fromEntries(formData);
try {
// Server-side validation
const validatedData = await validateOnServer(data);
return { success: true, data: validatedData };
} catch (error) {
return { success: false, error: error.message };
}
}
For React Hook Form:
// Integrate with Zod for enhanced validation
import { zodResolver } from '@hookform/resolvers/zod';
import * as z from 'zod';
const schema = z.object({
username: z.string().min(3, 'Username must be at least 3 characters'),
password: z.string().min(8, 'Password must be at least 8 characters')
});
function Form() {
const { register, handleSubmit } = useForm({
resolver: zodResolver(schema)
});
}
Conclusion
The choice between useActionState
and React Hook Form ultimately depends on your specific needs and constraints. If you're building simple forms with server-side processing, useActionState
might be sufficient. However, for complex forms requiring immediate validation and enhanced user feedback, React Hook Form provides a more comprehensive solution.
Remember that these tools aren't mutually exclusive - you can use both in different parts of your application based on specific requirements. As one experienced developer pointed out, "It's not about choosing one over the other permanently, but about picking the right tool for each specific use case."
The key is to understand your requirements thoroughly and choose the solution that best serves your users while maintaining code quality and developer productivity. Whether you opt for the native simplicity of useActionState
or the robust features of React Hook Form, ensure your choice aligns with your project's architecture and performance goals.