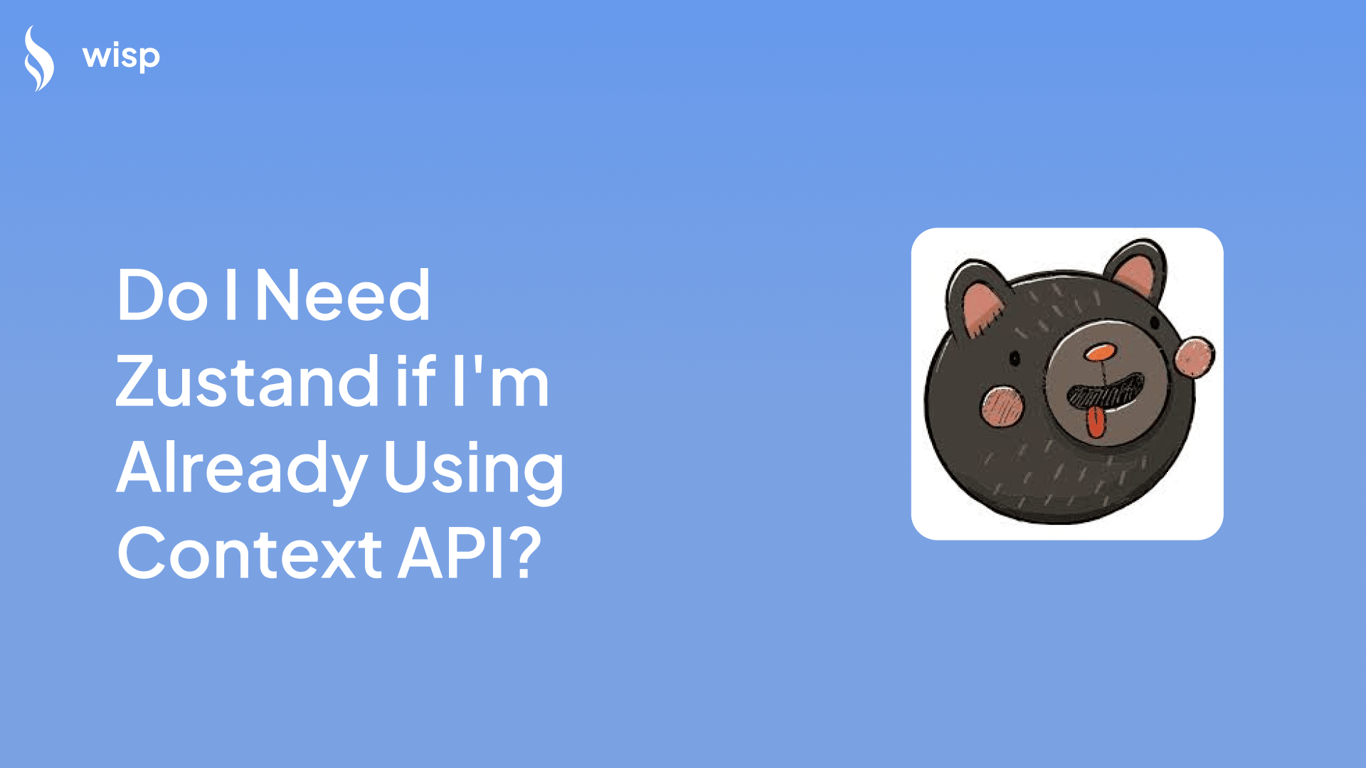
You've been using React's Context API for state management in your project, but lately you've been hearing a lot about Zustand. Now you're wondering if you should make the switch, or if you even need Zustand at all. You're not alone in this confusion - many developers struggle with choosing between these two approaches for state management.
"I'm still confused about when I should use store vs context since they both can do similar things," is a common sentiment echoed across the React community. The good news is, understanding when to use each tool can significantly improve your application's performance and development experience.
Understanding the Core Differences
Let's start by breaking down what makes these tools different and why you might want to consider using Zustand alongside (or instead of) Context API.
The Context API Approach
React's Context API is built into React itself and serves a specific purpose: avoiding prop drilling in parent-to-child component hierarchies. It's like creating a direct tunnel between components that need to share data, bypassing all the components in between.
However, there's a crucial misconception here. As one experienced developer points out, "Context API is not for global state. It's for avoiding prop drilling in a well-defined hierarchy of parent-to-child components." This distinction is important because using Context API for global state management can lead to performance issues.
Consider this common pain point:
// Using Context API
const MyContext = createContext();
const MyProvider = ({ children }) => {
const [state, setState] = useState({
user: null,
theme: 'light',
notifications: []
});
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
};
While this works, every component consuming this context will re-render whenever any part of the state changes, even if they only care about a specific piece of the state.
Enter Zustand
Zustand, on the other hand, was built specifically for state management. It provides a simpler, more performant approach to handling global state. Here's what the same state management looks like with Zustand:
import create from 'zustand';
const useStore = create((set) => ({
user: null,
theme: 'light',
notifications: [],
setUser: (user) => set({ user }),
setTheme: (theme) => set({ theme }),
setNotifications: (notifications) => set({ notifications })
}));
The key difference? Components using this store will only re-render when their specific subscribed state changes. Plus, you don't need to wrap your app in any providers - Zustand handles all of that for you.
When Context API Shines
Context API is perfect for certain scenarios:
Theme Management: When you need to provide theme information to deeply nested components
User Preferences: For settings that don't change frequently
Localization Data: When providing translation strings throughout your app
Authentication State: For sharing user authentication status
These use cases share a common trait: they involve relatively static data that doesn't update frequently.
When Zustand Takes the Lead
Zustand becomes particularly valuable when you're dealing with:
Frequently Updating State: When your state changes often and you need to prevent unnecessary re-renders
Complex State Logic: When you need to manage multiple state updates with side effects
Performance Critical Applications: When every render counts and you need fine-grained control over updates
Global State Management: When you need a single source of truth for your application state
Here's a real-world example that demonstrates why developers often switch to Zustand:
// Before: With Context API
const useNotifications = () => {
const context = useContext(NotificationContext);
if (!context) {
throw new Error('useNotifications must be used within NotificationProvider');
}
return context;
};
// After: With Zustand
const useNotifications = create((set) => ({
notifications: [],
addNotification: (notification) =>
set((state) => ({
notifications: [...state.notifications, notification]
})),
removeNotification: (id) =>
set((state) => ({
notifications: state.notifications.filter(n => n.id !== id)
}))
}));
The Zustand version is not only more concise but also more performant, as it only triggers re-renders in components that specifically subscribe to the notifications state.
Common Pitfalls to Avoid
Overusing Global State
One of the most common mistakes developers make is overusing global state management. As one developer shares, "I recently started contributing to a project that's been in development for a while and is almost exclusively using global state management for everything. I'm talking checkbox state, text input state, modal open state, etc."
This is a classic anti-pattern. Not every piece of state needs to be global. Here's a quick guide on what should stay local:
Form input states
UI component states (like modal open/close)
Temporary data that doesn't need to be shared
Component-specific loading states
The Performance Impact
Context API's performance limitations become apparent when you have:
Frequent state updates
Many context consumers
Complex state structures
Here's a performance comparison example:
// Context API - All consumers re-render when any part of the state changes
const UserContext = createContext();
const UserProvider = ({ children }) => {
const [user, setUser] = useState(null);
const [preferences, setPreferences] = useState({});
return (
<UserContext.Provider value={{ user, preferences, setUser, setPreferences }}>
{children}
</UserContext.Provider>
);
};
// Zustand - Only affected components re-render
const useUserStore = create((set) => ({
user: null,
preferences: {},
setUser: (user) => set({ user }),
setPreferences: (preferences) => set({ preferences })
}));
// Component only interested in user data
const UserProfile = () => {
// Only re-renders when user changes
const user = useUserStore((state) => state.user);
return <div>{user.name}</div>;
};
Making the Transition
If you're considering moving from Context API to Zustand, here's a practical approach:
Start Small: Begin by moving frequently changing global state to Zustand
Keep Context for Static Data: Maintain Context API for truly static, widely-shared data
Measure Performance: Use React DevTools to monitor re-renders and performance improvements
Making the Right Choice
The decision between Zustand and Context API doesn't have to be an either/or choice. Many successful React applications use both, leveraging each tool's strengths:
Use Context API When:
You need to avoid prop drilling in a well-defined component hierarchy
The state changes infrequently
You're dealing with static data that needs to be accessible throughout your app
You want to stick with React's built-in solutions
Use Zustand When:
You need efficient global state management
Your state updates frequently
Performance is a critical concern
You want a simpler API with less boilerplate
Conclusion
As one developer who made the switch notes, "I'm now finally learning Zustand after getting frustrated with React Context, especially with all the cumbersome code that it requires." This sentiment reflects a common journey in the React ecosystem - starting with Context API and gradually adopting Zustand for more complex state management needs.
Remember:
Context API and Zustand serve different primary purposes
They can coexist in the same application
Choose based on your specific use case, not just because one is newer or more popular
Consider the performance implications of your choice
By understanding these tools' strengths and limitations, you can make an informed decision that best serves your application's needs. Whether you choose to use Context API, Zustand, or both, the key is to use them appropriately for their intended purposes.
For more detailed information and examples, you can refer to: