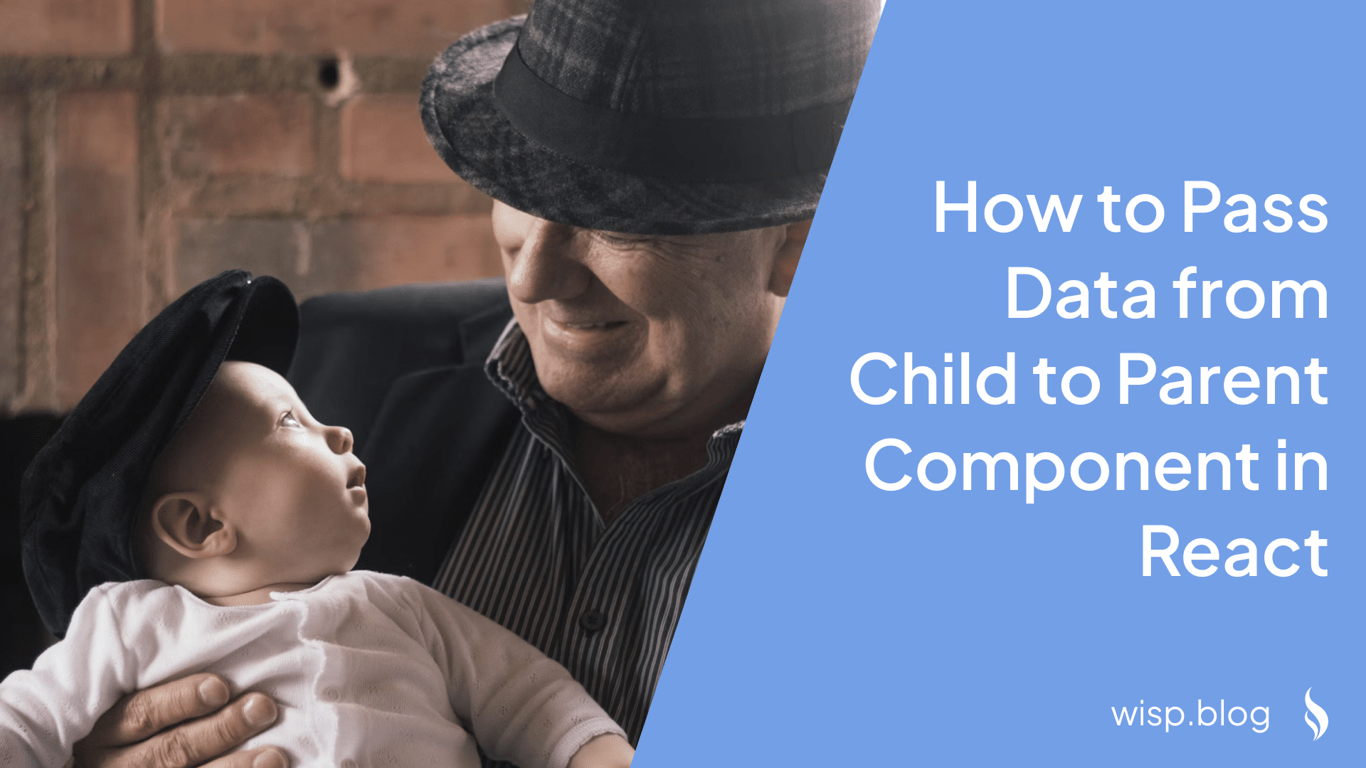
You've set up your React components, and everything seems to be working fine with data flowing down from parent to child. But now you need to send data back up from a child component to its parent, and suddenly you're stuck. If you're feeling frustrated or questioning your abilities - don't worry, you're not alone.
Many developers, especially those new to React, find this concept challenging. As one developer shared on Reddit, "I've been watching lectures for 2 weeks now and I simply don't understand how this works." The good news is that once you grasp the core concepts, child-to-parent communication in React becomes second nature.
In this comprehensive guide, we'll explore various methods to pass data from child to parent components, along with the crucial questions you should ask before choosing an approach. Whether you're preparing for interviews or building your next React application, this knowledge will prove invaluable.
Understanding the Basics: The React Data Flow
Before diving into specific solutions, it's essential to understand React's fundamental principle: data flows in one direction, from parent to child. This unidirectional data flow is intentional and helps maintain predictable state management in your applications.
However, there are several well-established patterns for sending data back up the component tree. Let's explore each method, starting with the most common approach.
Method 1: Callback Functions - The Classic Approach
The most straightforward way to pass data from child to parent is through callback functions. Here's how it works:
Define a function in the parent component
Pass this function as a prop to the child component
Call the function from the child component with the data as an argument
Here's a practical example:
// Parent Component
function ParentComponent() {
const [message, setMessage] = useState('');
const handleChildData = (data) => {
setMessage(data);
};
return (
<div>
<h1>Message from child: {message}</h1>
<ChildComponent onDataSend={handleChildData} />
</div>
);
}
// Child Component
function ChildComponent({ onDataSend }) {
const sendDataToParent = () => {
onDataSend('Hello from child!');
};
return (
<button onClick={sendDataToParent}>
Send Message to Parent
</button>
);
}
This pattern is particularly useful when you need to:
Send data on specific events (like button clicks)
Update parent state based on child component actions
Handle form submissions from child components
Method 2: Lifting State Up - The React Way
"Lifting state up" is a fundamental React pattern that's often overlooked but incredibly powerful. Instead of trying to send data up, you maintain the state in the parent component and pass down both the state value and the setter function.
Here's how to implement this pattern:
// Parent Component
function ParentComponent() {
const [inputValue, setInputValue] = useState('');
return (
<div>
<h2>Current Value: {inputValue}</h2>
<ChildComponent
value={inputValue}
setValue={setInputValue}
/>
</div>
);
}
// Child Component
function ChildComponent({ value, setValue }) {
return (
<input
type="text"
value={value}
onChange={(e) => setValue(e.target.value)}
/>
);
}
This approach has several advantages:
Maintains a single source of truth
Reduces complexity in state management
Makes it easier to share state between sibling components
Follows React's recommended patterns for state management
Method 3: Context API - The Global Solution
When you need to share data between components that aren't directly related (parent-child), or when you want to avoid prop drilling, the Context API provides an elegant solution:
// Create a context
const DataContext = React.createContext();
// Parent/Provider Component
function ParentComponent() {
const [sharedData, setSharedData] = useState('');
return (
<DataContext.Provider value={{ sharedData, setSharedData }}>
<div>
<h2>Shared Data: {sharedData}</h2>
<ChildComponent />
</div>
</DataContext.Provider>
);
}
// Child Component
function ChildComponent() {
const { setSharedData } = useContext(DataContext);
return (
<button onClick={() => setSharedData('Updated from child!')}>
Update Shared Data
</button>
);
}
The Context API is particularly useful when:
Multiple components need access to the same data
You want to avoid passing props through intermediate components
You need a lightweight alternative to state management libraries
Method 4: Refs and forwardRef - The Direct Line
Sometimes you need a more direct way to interact with child components. React's useRef
and forwardRef
provide this capability:
// Parent Component
function ParentComponent() {
const childRef = useRef();
const handleParentButtonClick = () => {
// Directly call child's method
childRef.current.childMethod();
};
return (
<div>
<button onClick={handleParentButtonClick}>
Call Child Method
</button>
<ChildComponent ref={childRef} />
</div>
);
}
// Child Component
const ChildComponent = forwardRef((props, ref) => {
const childMethod = () => {
console.log('Method called from parent!');
};
// Expose the method to parent
useImperativeHandle(ref, () => ({
childMethod
}));
return <div>Child Component</div>;
});
This approach is useful for:
Triggering imperative actions in child components
Accessing child DOM nodes directly
Managing focus, text selection, or animations
Important Questions to Consider
Before choosing a method for child-to-parent communication, ask yourself these crucial questions:
1. What is the scope of the data?
Is it needed only by the immediate parent?
Do multiple components need access to this data?
Will the data need to be shared with sibling components?
2. How frequently will the data change?
Are we dealing with frequent updates?
Do we need to optimize for performance?
Should we implement debouncing or throttling?
3. What is the complexity of the data?
Is it a simple value or complex object?
Do we need to maintain a history of changes?
Are there derived values that need to be calculated?
Best Practices and Common Pitfalls
Best Practices
Keep It Simple
Start with callback functions for simple parent-child communication
Only introduce Context or state management libraries when necessary
Use the lifting state up pattern for closely related components
Optimize Performance
// Use React.memo to prevent unnecessary re-renders const ChildComponent = React.memo(({ onDataSend }) => { // Component logic here }); // Use useCallback for function props const handleChildData = useCallback((data) => { setMessage(data); }, []); // Dependencies array
Maintain Clear Communication Patterns
Document your component communication strategy
Use consistent naming conventions for props and handlers
Keep data flow predictable and traceable
Common Pitfalls to Avoid
Prop Drilling
Don't pass props through multiple levels of components unnecessarily
Consider Context API or state management libraries for deep component trees
Overusing Context
Don't use Context for data that only needs to be passed down one or two levels
Remember that Context changes can trigger re-renders in all consuming components
Mutating State Directly
// Wrong const handleChange = (data) => { state.value = data; // Direct mutation }; // Correct const handleChange = (data) => { setState(prevState => ({ ...prevState, value: data })); };
Conclusion
Understanding how to pass data from child to parent components is crucial for building robust React applications. While it might seem daunting at first, as many developers have expressed in online communities, it becomes more intuitive with practice.
Remember:
Start with the simplest solution that meets your needs
Consider the scope and frequency of data updates
Choose the appropriate method based on your specific use case
Pay attention to performance implications
Follow React's best practices and patterns
Whether you're preparing for interviews or building production applications, mastering these patterns will make you a more effective React developer. Don't feel discouraged if it takes time to grasp these concepts - every developer has been through this learning curve.
Keep practicing, experimenting with different approaches, and most importantly, don't hesitate to ask questions when you're stuck. The React community is supportive and always willing to help fellow developers grow.