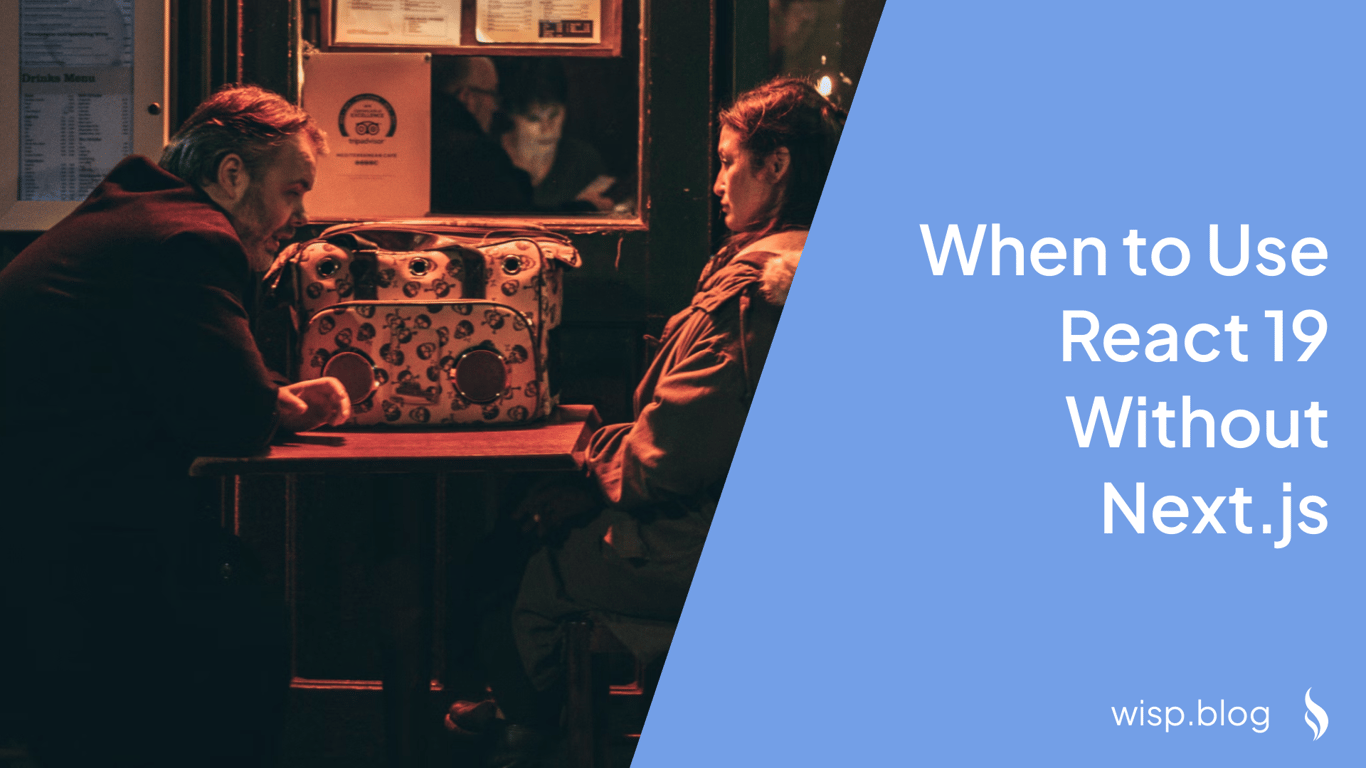
You're starting a new React project and feeling the pressure to use Next.js because "that's what everyone uses nowadays." But is Next.js really necessary for your specific use case? With React 19's powerful new features, you might be surprised to learn that vanilla React could be all you need.
The Rise of React 19
React 19 introduces game-changing features that significantly enhance its standalone capabilities. The new React Compiler can potentially double your application's performance while simplifying state management. Server Components now allow server-side processing without relying on Next.js, and new hooks like useOptimistic
, useFormStatus
, and useFormState
make managing UI states more intuitive than ever.
As one developer noted on Reddit, "When I don't need SSR/SEO, that's the problem that Next.js solves." This sentiment echoes throughout the developer community, highlighting that Next.js might be overkill for many projects.
When React 19 Alone Makes Sense
1. Client-Side Focused Applications
If your application primarily relies on client-side rendering and doesn't require extensive SEO optimization, React 19 provides everything you need. As highlighted by developers in this discussion, "My team uses react + vite + redux toolkit and haven't seen reason or need to switch to nextJS."
Common examples include:
Internal dashboards
Admin panels
SaaS applications
Data visualization tools
Interactive web applications
2. Cost-Conscious Projects
For backend-heavy applications, using Next.js might lead to unnecessary server costs. A developer pointed out, "When you don't want to pay for more compute, perhaps- especially if you're backend heavy instead of frontend heavy." React 19's improved client-side capabilities can help you build powerful applications while keeping server costs down.
3. Simple, Appearance-Oriented Projects
Reddit discussions consistently mention that "For simple, appearance-oriented pages (e.g. portfolios, landing pages etc) you do not need NextJS." React 19's enhanced features provide everything needed for:
Portfolio websites
Landing pages
Marketing sites
Product showcases
Event websites
4. Learning and Skill Development
Many developers feel overwhelmed by the pressure to learn Next.js immediately. As expressed in this thread, there's uncertainty about "the necessity of deep React knowledge in the context of modern full stack development."
Starting with React 19 allows you to:
Master fundamental React concepts
Understand component lifecycle
Learn state management patterns
Build a strong foundation before adding framework complexity
Addressing Common Concerns
Library Compatibility
One significant concern with React 19 is library compatibility. As one developer mentioned, "a lot of libraries are not ready to use with React 19, or are in experimental mode themselves." However, this is a temporary situation that will resolve as the ecosystem catches up.
State Management
While Next.js provides certain conveniences, React 19's native capabilities are more than sufficient for most state management needs. You can effectively manage state using:
React's built-in Context API for global state
The new hooks in React 19 for local state management
Third-party solutions like Redux Toolkit when needed
Routing Solutions
The lack of built-in routing in React is often cited as a limitation. However, as recommended by developers, React Router provides a robust solution that can be set up in a single file and manages page navigation effectively.
Making the Decision: React 19 vs Next.js
To help you decide if React 19 alone is right for your project, consider these questions:
SEO Requirements
Do you need extensive SEO optimization?
Is server-side rendering crucial for your content?
Application Type
Is it an internal tool or public-facing application?
How important is initial load time?
Development Resources
What's your team's expertise level?
Do you have the backend infrastructure to support SSR if needed?
Cost Considerations
Are you working with limited server resources?
Is your application backend-heavy?
Best Practices for React 19 Projects
When building with React 19 without Next.js, follow these best practices to maximize your application's potential:
Leverage the React Compiler
Enable the compiler using
eslint-plugin-react-compiler@experimental
Take advantage of automatic optimizations for better performance
Implement Proper Code Splitting
Use React.lazy() for component-level code splitting
Implement route-based splitting for better initial load times
Optimize State Management
Utilize React 19's new hooks effectively
Implement Context API strategically for global state
Consider Redux Toolkit for complex state requirements
Focus on Performance
Implement proper memoization using
useMemo
anduseCallback
Optimize asset loading and management
Monitor and optimize re-renders
Conclusion
As one developer wisely stated, "There's tons of apps in production out there that don't use Next but just use plain React." React 19's enhanced capabilities make it an even more viable option for many projects.
The decision to use React 19 without Next.js should be based on your specific project requirements rather than following trends. For many applications, especially those focused on client-side rendering or requiring minimal server-side functionality, React 19 provides all the tools needed for success.
Remember, as noted by experienced developers, "Every tool has its purpose." Sometimes, the simpler solution is the better one. React 19's improvements in performance, developer experience, and built-in capabilities make it a strong choice for modern web development, with or without Next.js.