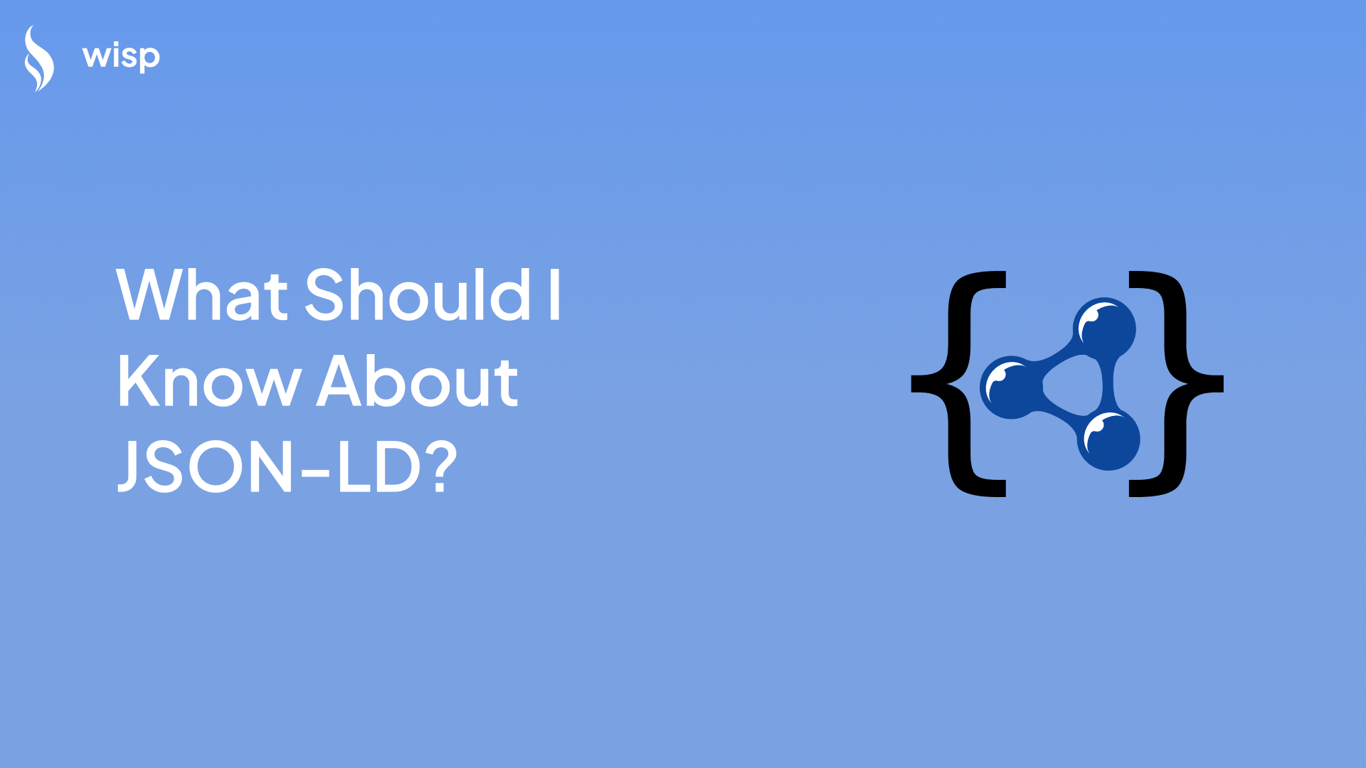
As a web developer in today's rapidly evolving digital landscape, you've likely encountered the challenge of making your data more accessible and meaningful to both machines and humans. You might have struggled with hard-coded URLs creating brittle client-server relationships, or found yourself frustrated with complex schema markup methods. This is where JSON-LD (JavaScript Object Notation for Linked Data) comes into play.
Understanding JSON-LD and Its Significance
JSON-LD represents a significant evolution in how we structure and share data on the web. While only 25.1% of websites currently use JSON-LD structured data, its importance is growing rapidly, especially as we move towards a more semantic web (Web 3.0).
Think of JSON-LD as your translator between the human-readable JSON format you're familiar with and the machine-readable linked data that search engines and other automated systems crave. It solves a critical problem in modern web development: the strong coupling between clients and servers in traditional JSON APIs that often limits flexibility and creates maintenance headaches.
The Core Components of JSON-LD
At its heart, JSON-LD builds upon the JSON format you already know and love, adding a few special keys that transform regular JSON into linked data:
{
"@context": "https://schema.org",
"@type": "Person",
"name": "John Doe",
"jobTitle": "Software Engineer",
"url": "https://example.com/john"
}
Let's break down these essential components:
@context: This is your data's vocabulary definition. It tells machines where to look up the meaning of your properties. Think of it as a dictionary that defines your terms.
@type: This specifies what kind of thing you're describing. It's like declaring a class in programming - it tells machines "this is a Person" or "this is a Product."
Why JSON-LD Matters for Modern Web Development
Solving the API Flexibility Challenge
One of the biggest pain points in web development is creating APIs that are both simple to use and flexible enough to evolve over time. As noted in the W3C JSON-LD Best Practices, developers often find themselves "designed into a corner" with traditional APIs, leading to frustrating version changes that require extensive client-side updates.
JSON-LD addresses this by:
Decoupling data representation from specific URLs
Allowing for graceful evolution of APIs without breaking changes
Reducing the need for version-specific client code
Enhanced SEO Benefits
If you've ever struggled with implementing schema markup using RDFa or microdata, you'll appreciate JSON-LD's approach. Google has explicitly stated their preference for JSON-LD as the recommended format for structured data, and for good reason:
Cleaner Implementation: Unlike traditional methods, JSON-LD doesn't require you to modify your HTML markup, making it easier to maintain and update.
Rich Snippets: JSON-LD enables rich snippets in Google's Knowledge Graph, potentially leading to:
Enhanced visibility in search results
Brand logo display next to search results
Review stars for products
Better click-through rates
Separation of Concerns: Your structured data remains separate from your presentation markup, making it easier to manage and update.
Practical Implementation Guide
Getting Started with JSON-LD
Here's a practical example of implementing JSON-LD for a business website:
{
"@context": "https://schema.org",
"@type": "LocalBusiness",
"name": "Tech Solutions Inc",
"description": "Professional web development services",
"address": {
"@type": "PostalAddress",
"streetAddress": "123 Code Street",
"addressLocality": "Tech City",
"addressRegion": "TC",
"postalCode": "12345",
"addressCountry": "US"
},
"telephone": "+1-234-567-8900",
"url": "https://techsolutions.example.com"
}
Best Practices for Implementation
When implementing JSON-LD, keep these key practices in mind:
Placement in HTML
<head> <script type="application/ld+json"> { "@context": "https://schema.org", "@type": "WebPage", "name": "Your Page Title" } </script> </head>
Validation and Testing
Always validate your JSON-LD using Google's Structured Data Testing Tool
Test across different page types to ensure consistency
Monitor Google Search Console for structured data errors
Dynamic Content Handling When dealing with dynamic content, ensure your JSON-LD stays synchronized:
const generateProductLD = (product) => { return { "@context": "https://schema.org", "@type": "Product", "name": product.name, "description": product.description, "price": product.price, "currency": "USD" }; };
Common Challenges and Solutions
1. Parsing Errors
One of the most common frustrations developers face is JSON-LD parsing errors. As seen in recent discussions, these often manifest as syntax issues like missing brackets or incorrect formatting.
Solution:
// Before sending to parser, validate JSON structure
try {
JSON.parse(jsonldString);
// Valid JSON structure
} catch (e) {
console.error("Invalid JSON structure:", e);
}
2. Multiple Type Definitions
Sometimes you need to represent entities with multiple types:
{
"@context": "https://schema.org",
"@type": ["Person", "Organization"],
"name": "John Smith",
"founder": {
"@type": "Organization",
"name": "Example Corp"
}
}
3. Handling Complex Relationships
When dealing with complex data relationships, use the @id
property to create references:
{
"@context": "https://schema.org",
"@graph": [
{
"@type": "Person",
"@id": "#jane",
"name": "Jane Doe",
"worksFor": {"@id": "#company"}
},
{
"@type": "Organization",
"@id": "#company",
"name": "Tech Corp",
"employee": {"@id": "#jane"}
}
]
}
Advanced Features and Techniques
1. Context Management
For larger applications, consider creating a custom context:
{
"@context": {
"@vocab": "https://schema.org/",
"my": "http://my.company.com/terms/",
"customField": "my:customField"
}
}
2. Internationalization Support
JSON-LD provides excellent support for multiple languages:
{
"@context": "https://schema.org",
"@type": "Product",
"name": {
"@value": "Computer",
"@language": "en"
},
"description": [
{
"@value": "A high-performance laptop",
"@language": "en"
},
{
"@value": "Un ordinateur portable haute performance",
"@language": "fr"
}
]
}
Future-Proofing Your Implementation
Staying Current with Standards
The JSON-LD specification continues to evolve, with version 1.1 bringing new features and improvements. To ensure your implementation remains robust:
Monitor W3C Updates
Regular check the official JSON-LD specifications
Subscribe to relevant working group communications
Implement Progressive Enhancement
// Check for JSON-LD support if (document.head.querySelector('script[type="application/ld+json"]')) { // Enhanced features available } else { // Fallback implementation }
Plan for Scalability
Design your JSON-LD structure to accommodate future data needs
Use modular approaches to context management
Implement proper caching strategies for context files
Conclusion
JSON-LD represents a crucial evolution in web development, bridging the gap between human-readable JSON and machine-understandable linked data. While only a quarter of websites currently utilize JSON-LD structured data, its adoption is growing rapidly due to its clear benefits:
Improved SEO through better structured data implementation
Enhanced API flexibility and maintainability
Cleaner separation of concerns in data representation
Future-proof approach to semantic web integration
As you implement JSON-LD in your projects, remember to:
Start with proper validation and testing
Follow best practices for implementation
Stay current with evolving standards
Plan for scalability and future needs
By mastering JSON-LD, you're not just improving your current projects – you're preparing for the future of the semantic web and ensuring your applications remain robust and maintainable in the long term.
For more information and ongoing updates, consider following the W3C JSON-LD Working Group and participating in the developer community discussions around structured data implementation.