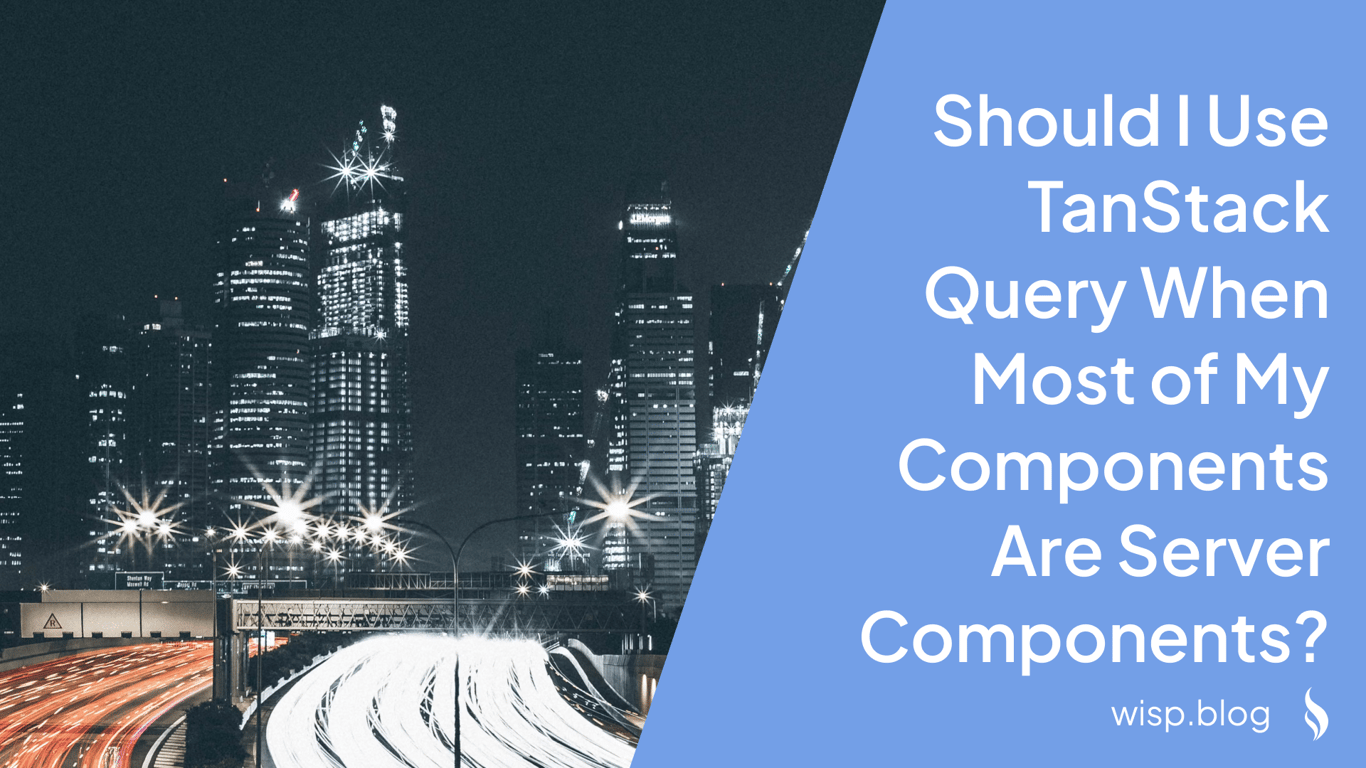
You've embraced React Server Components in your application, leveraging their powerful server-side rendering capabilities. But now you're wondering: "Isn't most of the added value lost if we have server components?" This is a common concern echoing through the developer community, especially when considering whether to integrate TanStack Query into a server-component-heavy architecture.
The short answer? TanStack Query still offers significant value, even in applications predominantly using server components. Let's explore why and when you should consider using it.
Understanding the Dilemma
Modern React applications often combine both server and client components, creating a hybrid architecture that can sometimes feel complex to manage. While server components excel at initial data fetching and rendering, real-world applications frequently require more dynamic data interactions that happen after the initial page load.
Consider these common scenarios:
Opening a dialog to view detailed entity information
Implementing real-time updates for dynamic content
Managing complex state across multiple components
Handling non-serializable data from the server
Maintaining consistent URL states during pagination or filtering
These scenarios often demand more sophisticated state management than what server components alone can provide. This is where TanStack Query shines, even in a server-component-dominated application.
The Power of TanStack Query
TanStack Query isn't just another state management library - it's specifically designed to handle the complexities of server state in your application. Here's what makes it particularly valuable:
Robust Caching System: TanStack Query provides an intelligent caching mechanism that can significantly improve your application's performance and user experience. Even with server components handling the initial data fetch, subsequent interactions can benefit from this caching layer.
Automatic Background Updates: The library manages background data refreshing and revalidation, ensuring your UI stays in sync with server data without manual intervention.
Complex State Management: It handles loading, error, and success states automatically, reducing boilerplate code and making your components cleaner and more maintainable.
Optimistic Updates: When modifying data, TanStack Query allows you to update the UI immediately while handling the server communication in the background, creating a more responsive user experience.
Real-World Use Cases
Let's explore specific scenarios where TanStack Query proves invaluable, even in applications heavily utilizing server components.
1. Dynamic Dialog Content
When you need to open a dialog to view details about an entity, server components alone might not provide the best user experience. Here's how TanStack Query can help:
function EntityDetailsDialog({ entityId }) {
const { data, isLoading, error } = useQuery({
queryKey: ['entity', entityId],
queryFn: () => fetchEntityDetails(entityId)
});
if (isLoading) return <LoadingSpinner />;
if (error) return <ErrorMessage error={error} />;
return (
<Dialog>
<DialogContent>
<h2>{data.title}</h2>
<p>{data.description}</p>
</DialogContent>
</Dialog>
);
}
This approach provides smooth loading states and error handling while maintaining a responsive user interface.
2. Real-Time Updates
Many developers express the need to handle real-time data updates efficiently. As one developer noted in a Reddit discussion: "I just use it for fetching client side, but I'd like to go back through and use prefetch on the server because there are some instances where I need it server and client (mostly real-time stuff)."
TanStack Query makes this easier with its refetching capabilities:
function LiveDataComponent() {
const { data } = useQuery({
queryKey: ['liveData'],
queryFn: fetchLiveData,
refetchInterval: 5000 // Refetch every 5 seconds
});
return <div>{/* Render live data */}</div>;
}
3. URL State Management
For applications requiring pagination or filtering with URL state preservation, TanStack Query integrates seamlessly with URL parameters:
function ProductList() {
const [searchParams] = useSearchParams();
const page = searchParams.get('page') || '1';
const { data, isLoading } = useQuery({
queryKey: ['products', page],
queryFn: () => fetchProducts(page),
keepPreviousData: true // Smooth pagination experience
});
return (
<div>
{/* Render product list with pagination */}
</div>
);
}
Best Practices for Integration
When combining TanStack Query with server components, following these best practices will help you maximize the benefits while avoiding common pitfalls:
1. Selective Implementation
Don't feel pressured to use TanStack Query for every data fetching operation. As one developer wisely noted, "If it's possible to get the data on the server side, this should be done, without the help of Tanstack Query (except for prefetching)." Use it strategically where it adds the most value:
Dynamic data requirements
Client-side interactions
Real-time updates
Complex caching needs
2. Leverage Prefetching
TanStack Query's prefetching capabilities can bridge the gap between server and client components:
// In your server component
await queryClient.prefetchQuery({
queryKey: ['data'],
queryFn: () => fetchData()
});
// In your client component
function ClientComponent() {
const { data } = useQuery({
queryKey: ['data'],
queryFn: () => fetchData()
});
return <div>{/* Render data */}</div>;
}
3. Handle Non-Serializable Data
When dealing with data that can't be serialized for server components, TanStack Query provides an elegant solution:
function DownloadComponent() {
const { data } = useQuery({
queryKey: ['binaryData'],
queryFn: () => fetchBinaryData(),
enabled: false // Only fetch when needed
});
return (
<button onClick={() => queryClient.fetchQuery(['binaryData'])}>
Download File
</button>
);
}
4. Optimize Performance
Take advantage of TanStack Query's built-in optimization features:
const queryClient = new QueryClient({
defaultOptions: {
queries: {
staleTime: 1000 * 60 * 5, // Data considered fresh for 5 minutes
cacheTime: 1000 * 60 * 30, // Cache persists for 30 minutes
refetchOnWindowFocus: false // Disable automatic refetching on window focus
}
}
});
Making the Decision
When deciding whether to implement TanStack Query in your server-component-heavy application, consider these key factors:
1. Application Complexity
If you're building a simple application with minimal client-side interactions, you might not need TanStack Query. As one developer mentioned, "I haven't worked on an app big/elaborate enough to benefit from react-query/tanstack-query." However, as your application grows and requires more dynamic data handling, TanStack Query's benefits become more apparent.
2. User Experience Requirements
Consider implementing TanStack Query if your application needs:
Instant UI updates with optimistic mutations
Sophisticated loading and error states
Background data synchronization
Complex caching strategies
3. Development Team Experience
TanStack Query can significantly improve code maintainability and reduce boilerplate. As noted in community discussions, "It helps in caching and clean code, as it gives all the state (loading error pending) that is helpful for me."
Conclusion
While server components have revolutionized how we approach data fetching in React applications, TanStack Query continues to provide significant value, even in server-component-heavy architectures. The key is understanding when and where to apply it strategically.
The library shines in scenarios requiring:
Complex client-side state management
Real-time data synchronization
Sophisticated caching strategies
Non-serializable data handling
Dynamic user interactions
Remember, it's not about choosing between server components and TanStack Query - it's about leveraging the strengths of both to build more robust, maintainable, and user-friendly applications.
Additional Resources
By thoughtfully combining server components with TanStack Query, you can create applications that offer the best of both worlds: excellent initial page loads and dynamic, responsive user interactions.