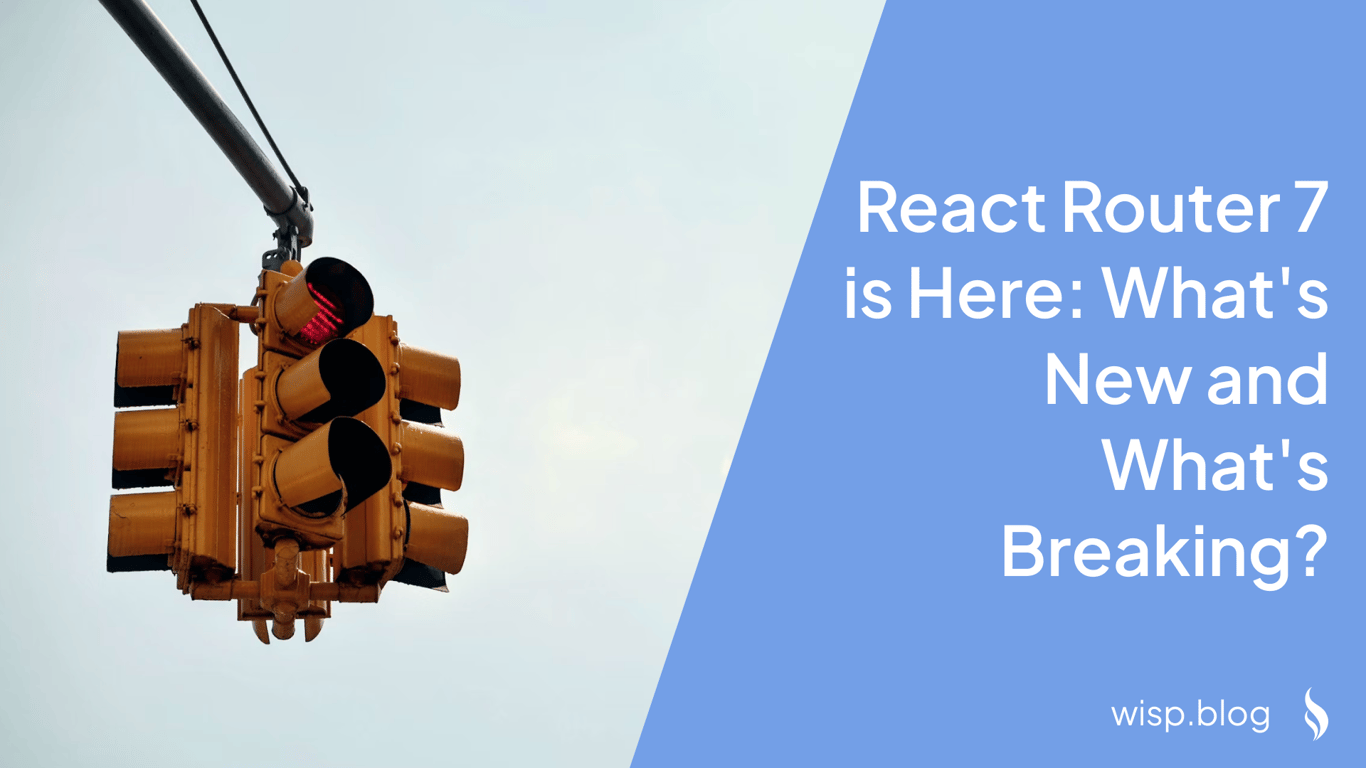
You've just heard about React Router 7's release, and if you're like many developers, you might be feeling a mix of curiosity and apprehension. Perhaps you're still recovering from the v5 to v6 migration headaches, or you're wondering if this update will bring another round of API rewrites that'll force you to refactor your routing logic yet again.
The developer community has been vocal about these concerns. As one developer put it on Reddit, "One of the only constants I've had in my entire programming career is that React Router is going to rewrite their API on every new release." This sentiment resonates with many who've been through multiple React Router migrations.
But here's the thing - while change can be challenging, React Router 7 brings some significant improvements that might make the upgrade worthwhile. Let's dive into what's new, what's breaking, and most importantly, how to make the transition as smooth as possible.
What's New in React Router 7?
1. Enhanced Type Safety
One of the most significant improvements in v7 is the enhanced type safety system. The Remix team has addressed a common pain point by introducing better TypeScript integration. Now you can enjoy more robust type checking and improved autocompletion support in your IDE.
// Before (React Router 6)
const loader = async ({ params }) => {
const user = await getUser(params.id);
return user;
};
// After (React Router 7)
const loader = async ({ params }: LoaderFunctionArgs) => {
const user = await getUser(params.id);
return json(user); // Type-safe response
};
2. Simplified Data Fetching
React Router 7 introduces a more intuitive approach to data fetching with improved loader and action APIs. This new implementation makes it easier to manage data dependencies and handle loading states:
// New data fetching pattern in v7
export const route = {
loader: async ({ params }) => {
const response = await fetch(`/api/users/${params.id}`);
return json(await response.json());
},
element: <UserProfile />
};
3. Improved Error Handling
Error handling gets a major upgrade in v7 with more granular control over error boundaries. You can now define error handling at the route level, making it easier to manage and debug issues:
const router = createBrowserRouter([
{
path: "/dashboard",
element: <Dashboard />,
errorElement: <DashboardError />,
children: [
{
path: "analytics",
element: <Analytics />,
errorElement: <AnalyticsError />, // Specific error handling
loader: analyticsLoader
}
]
}
]);
4. Better Suspense Integration
React Router 7 offers improved integration with React 18's Suspense feature, allowing for more elegant loading states and better user experience:
import { Suspense } from 'react';
import { Await, useLoaderData } from 'react-router-dom';
function ProductPage() {
const { product } = useLoaderData();
return (
<Suspense fallback={<ProductSkeleton />}>
<Await resolve={product}>
{(resolvedProduct) => <ProductDetails product={resolvedProduct} />}
</Await>
</Suspense>
);
}
Breaking Changes to Watch Out For
Before you upgrade, be aware of these significant breaking changes that might affect your application:
1. Response Objects are Now Required
// This won't work in v7
export async function loader() {
return { message: "Hello" };
}
// This is the correct way in v7
export async function loader() {
return json({ message: "Hello" });
}
2. Changes to Route Configuration
The route configuration syntax has been updated to be more explicit and type-safe:
// Old way (v6)
<Routes>
<Route path="/dashboard" element={<Dashboard />}>
<Route path="profile" element={<Profile />} />
</Route>
</Routes>
// New way (v7)
const router = createBrowserRouter([
{
path: "/dashboard",
element: <Dashboard />,
children: [
{
path: "profile",
element: <Profile />,
loader: profileLoader
}
]
}
]);
3. Updated Navigation API
The navigation API has been refined to be more intuitive and consistent:
// Old way (v6)
navigate('/dashboard');
// New way (v7)
navigation.navigate({
to: '/dashboard',
// New options available
preventScrollReset: true,
relative: 'path'
});
Migration Guide: Making the Switch
If you're ready to upgrade to React Router 7, here's a step-by-step guide to make the transition smoother:
1. Prepare Your Environment
First, ensure your project meets the minimum requirements:
# Update to React 18 if you haven't already
npm install react@18 react-dom@18
# Install React Router 7
npm install react-router-dom@7
2. Update Route Definitions
Convert your existing routes to the new format:
// src/router.tsx
import { createBrowserRouter } from 'react-router-dom';
export const router = createBrowserRouter([
{
path: '/',
element: <RootLayout />,
children: [
{
path: 'dashboard',
element: <Dashboard />,
loader: dashboardLoader,
errorElement: <DashboardError />
}
]
}
]);
3. Update Data Fetching Logic
Refactor your data fetching to use the new loader and action patterns:
// Before (v6)
function Dashboard() {
const [data, setData] = useState(null);
useEffect(() => {
fetchDashboardData().then(setData);
}, []);
return <div>{/* render data */}</div>;
}
// After (v7)
export async function loader() {
const data = await fetchDashboardData();
return json(data);
}
function Dashboard() {
const data = useLoaderData();
return <div>{/* render data */}</div>;
}
Should You Upgrade?
The decision to upgrade should be based on your specific needs and circumstances. Here are some considerations:
✅ Upgrade if:
You need better TypeScript support
You want improved data fetching patterns
You're starting a new project
You're already on v6 and want the latest features
⚠️ Wait if:
Your current setup is stable and working well
You're in the middle of a critical project phase
You haven't upgraded to v6 yet
You need time to thoroughly test the migration
Conclusion
React Router 7 represents a significant step forward in terms of type safety, data fetching, and developer experience. While some developers have reported performance concerns, the overall improvements in the API and functionality make it a compelling upgrade for many projects.
Remember to thoroughly test your application after upgrading, as breaking changes might affect your routing logic in unexpected ways. The React Router team has provided comprehensive migration documentation to help you through the process.
For those still hesitant about upgrading, it's perfectly acceptable to stay on v6 until you're ready to make the switch. As one developer wisely noted, "Choose the sucking you can tolerate" - sometimes stability is more valuable than having the latest features.