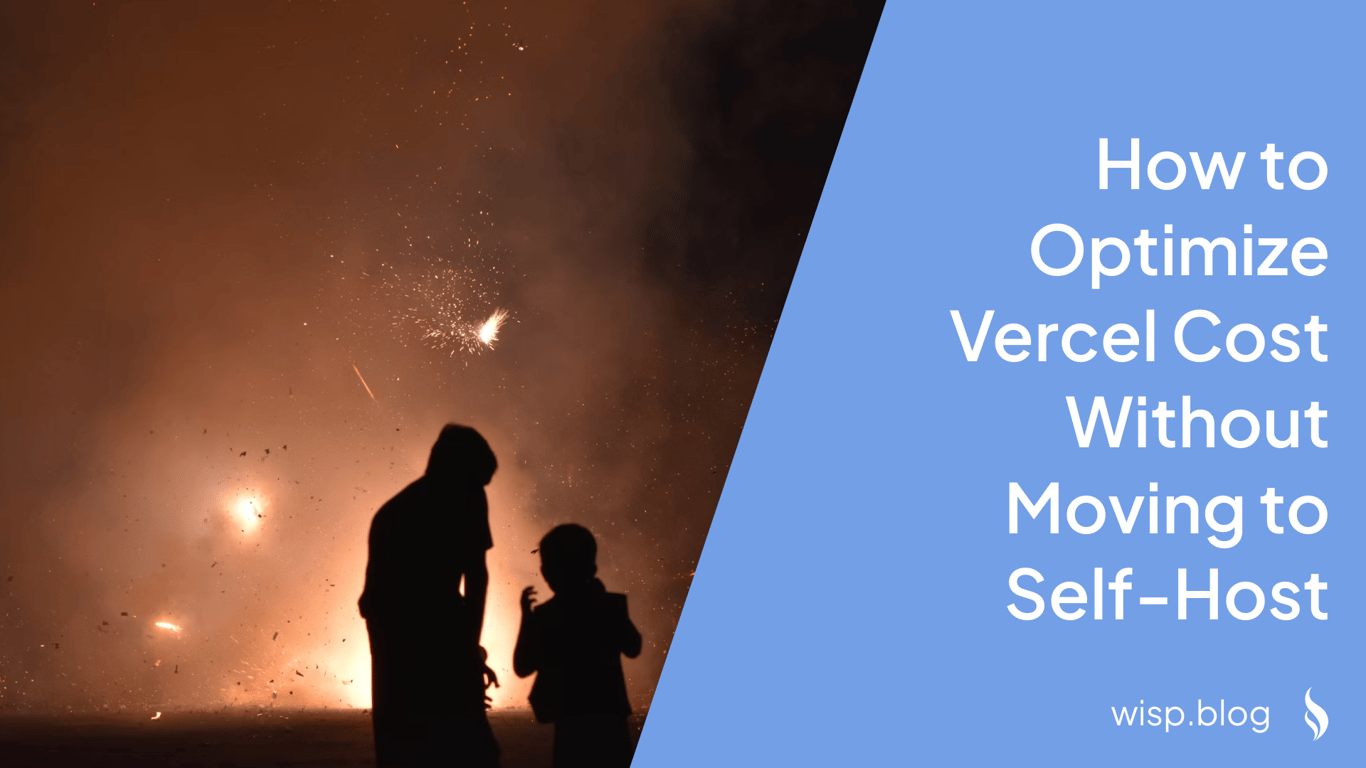
You've built an amazing Next.js application and deployed it on Vercel. Everything's running smoothly until you check your monthly bill - those costs are starting to add up fast! The bandwidth charges are climbing, and you're wondering if it's time to consider self-hosting. But before you go down that complex route, there are several effective strategies to optimize your Vercel costs while keeping all the platform benefits you love.
Understanding the Cost Challenge
If you're on Vercel's Pro plan ($20/user/month), you get 1TB of bandwidth included. However, once you exceed that limit, costs can skyrocket to $150++ per additional TB - a pricing structure that has caught many developers off guard. While self-hosting might seem tempting, it comes with its own set of challenges and hidden costs that could end up being more expensive in terms of time and resources.
Smart Image Optimization Strategies
One of the biggest factors affecting Vercel costs is image optimization. Here's how to handle it efficiently:
1. Use Pre-optimized Images
Instead of relying on Vercel's Image Optimization API, which can quickly consume your bandwidth allowance, consider pre-optimizing your images. Here's how:
Run your images through compression tools like TinyPNG/TinyJPG before uploading
Use the
unoptimized
attribute in Next.js Image component for these pre-optimized imagesStore different size variants of your images for different viewport sizes
2. Implement Custom Image Loaders
Consider using alternative image optimization services that can be more cost-effective. For example:
Set up WSrv as your image loader
Use Cloudinary's free tier for image optimization
Implement imgproxy for on-the-fly image processing
Here's a quick example of implementing a custom image loader:
const customLoader = ({ src, width, quality }) => {
return `https://wsrv.nl/?url=${src}&w=${width}&q=${quality || 75}`
}
<Image
loader={customLoader}
src="your-image-url.jpg"
alt="Description"
width={800}
height={600}
/>
Optimize Serverless Function Usage
Serverless functions are another significant cost factor on Vercel. Here's how to optimize them:
1. Implement Efficient Function Architecture
Combine multiple small functions into single endpoints where logical
Use server actions instead of API routes where possible - developers have reported significant cost savings with this approach
Implement proper error handling to prevent unnecessary function retries
2. Rate Limiting and Caching
Protect your serverless functions from abuse and reduce unnecessary invocations:
import rateLimit from 'express-rate-limit'
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
})
export default function handler(req, res) {
limiter(req, res, () => {
// Your function logic here
})
}
3. Leverage Edge Functions
Edge functions can be more cost-effective for certain operations:
Use them for simple transformations and routing logic
Implement geolocation-based redirects at the edge
Handle authentication and authorization at the edge
Build Optimization Strategies
Optimizing your build process can significantly impact both performance and costs:
1. Analyze and Reduce Bundle Size
Use the @next/bundle-analyzer
to identify large packages:
// next.config.js
const withBundleAnalyzer = require('@next/bundle-analyzer')({
enabled: process.env.ANALYZE === 'true'
})
module.exports = withBundleAnalyzer({
// your next config
})
2. Implement Code Splitting
Use dynamic imports for large components
Lazy load images and components below the fold
Split your code based on routes and features
Effective Caching Strategies
Proper caching can dramatically reduce your bandwidth usage and function invocations:
1. Implement Static Site Generation (SSG)
Maximize the use of static pages where possible:
// pages/blog/[slug].js
export async function getStaticProps({ params }) {
const post = await getPost(params.slug)
return {
props: { post },
revalidate: 3600 // Revalidate every hour
}
}
export async function getStaticPaths() {
const posts = await getAllPosts()
return {
paths: posts.map((post) => ({
params: { slug: post.slug }
})),
fallback: 'blocking'
}
}
2. Optimize Cache Headers
Set appropriate cache headers for your static assets:
// next.config.js
module.exports = {
async headers() {
return [
{
source: '/static/:path*',
headers: [
{
key: 'Cache-Control',
value: 'public, max-age=31536000, immutable'
}
]
}
]
}
}
3. Implement API Response Caching
Use Redis or similar solutions for API response caching:
import { Redis } from '@upstash/redis'
const redis = new Redis({
url: process.env.REDIS_URL,
token: process.env.REDIS_TOKEN,
})
export default async function handler(req, res) {
const cacheKey = `api:${req.url}`
const cached = await redis.get(cacheKey)
if (cached) {
return res.json(JSON.parse(cached))
}
const data = await fetchData()
await redis.set(cacheKey, JSON.stringify(data), { ex: 3600 })
res.json(data)
}
Monitoring and Optimization Tools
To maintain optimal cost efficiency, implement proper monitoring:
1. Set Up Usage Alerts
Create custom alerts in your Vercel dashboard to monitor:
Bandwidth usage
Function invocations
Build minutes
Deployment frequency
2. Regular Performance Audits
Conduct regular audits using tools like:
Lighthouse for performance metrics
Next.js Analytics for real-user monitoring
webpack-bundle-analyzer for bundle size analysis
Success Stories and Real-World Impact
Many teams have successfully optimized their Vercel costs without sacrificing performance. For instance, one team reported reducing their hosting costs by 80% by implementing server components and optimizing their deployment strategy. Another developer mentioned reducing their Amazon bill from $1,000 per month to just $40 by moving to Vercel and implementing these optimization techniques.
Conclusion
While Vercel's costs can seem daunting as your application scales, self-hosting isn't always the answer. By implementing these optimization strategies - from smart image handling to effective caching and monitoring - you can significantly reduce your Vercel costs while maintaining all the benefits of their platform.
Remember to:
Pre-optimize images and use custom image loaders
Implement efficient serverless function architecture
Maximize static generation and caching
Monitor usage and set up alerts
Regularly audit and optimize your application
Start with the strategies that align best with your current pain points and gradually implement others as needed. The key is to find the right balance between cost optimization and development efficiency.
For more detailed information and updates on cost optimization strategies, keep an eye on Vercel's official documentation and the active Next.js community discussions.